- Just saw your post as I was looking for a similar solution. I used the filter method you mentioned but failed to get desired results. The question is here Would you mind taking a look nad see if there's anything wrong with my code/method? IMO it shall be super simple albeit my being unable to make it work.
- This painting demonstration shows you how to complete a painting based on a strong pattern. Use the big shape pattern to make this process easier.
- Search for jobs related to Patterns cannot contain anything painted with a pattern or hire on the world's largest freelancing marketplace with 19m+ jobs. It's free to sign up and bid on jobs.
The options pattern uses classes to provide strongly-typed access to groups of related settings. When configuration settings are isolated by scenario into separate classes, the app adheres to two important software engineering principles:
- The Interface Segregation Principle (ISP) or Encapsulation: Scenarios (classes) that depend on configuration settings depend only on the configuration settings that they use.
- Separation of Concerns: Settings for different parts of the app aren't dependent or coupled to one another.
Options also provide a mechanism to validate configuration data. For more information, see the Options validation section.
Bind hierarchical configuration

Ravelry is a community site, an organizational tool, and a yarn & pattern database for knitters and crocheters.
The preferred way to read related configuration values is using the options pattern. The options pattern is possible through the IOptions<TOptions> interface, where the generic type parameter TOptions
is constrained to class
. The IOptions<TOptions>
can later be provided through dependency injection. For more information, see Dependency injection in .NET.
For example, to read the following configuration values:
Create the following TransientFaultHandlingOptions
class:
When using the options pattern, an options class:
- Must be non-abstract with a public parameterless constructor
- Contain public read-write properties to bind (fields are not bound)
The following code:
- Calls ConfigurationBinder.Bind to bind the
TransientFaultHandlingOptions
class to the'TransientFaultHandlingOptions'
section. - Displays the configuration data.
In the preceding code, changes to the JSON configuration file after the app has started are read.
ConfigurationBinder.Get<T>
binds and returns the specified type. ConfigurationBinder.Get<T>
may be more convenient than using ConfigurationBinder.Bind
. The following code shows how to use ConfigurationBinder.Get<T>
with the TransientFaultHandlingOptions
class:
In the preceding code, changes to the JSON configuration file after the app has started are read.
Important
The ConfigurationBinder class exposes several APIs, such as .Bind(object instance)
and .Get<T>()
that are not constrained to class
. When using any of the Options interfaces, you must adhere to aforementioned options class constraints.
An alternative approach when using the options pattern is to bind the 'TransientFaultHandlingOptions'
section and add it to the dependency injection service container. In the following code, TransientFaultHandlingOptions
is added to the service container with Configure and bound to configuration:
Tip
The key
parameter is the name of the configuration section to search for. It does not have to match the name of the type that represents it. For example, you could have a section named 'FaultHandling'
and it could be represented by the TransientFaultHandlingOptions
class. In this instance, you'd pass 'FaultHandling'
to the GetSection function instead. The nameof
operator is used as a convenience when the named section matches the type it corresponds to.
Using the preceding code, the following code reads the position options:
In the preceding code, changes to the JSON configuration file after the app has started are not read. To read changes after the app has started, use IOptionsSnapshot.
Options interfaces
IOptions<TOptions>:
- Does not support:
- Reading of configuration data after the app has started.
- Is registered as a Singleton and can be injected into any service lifetime.
IOptionsSnapshot<TOptions>:
- Is useful in scenarios where options should be recomputed on every injection resolution, in scoped or transient lifetimes. For more information, see Use IOptionsSnapshot to read updated data.
- Is registered as Scoped and therefore cannot be injected into a Singleton service.
- Supports named options
IOptionsMonitor<TOptions>:
- Is used to retrieve options and manage options notifications for
TOptions
instances. - Is registered as a Singleton and can be injected into any service lifetime.
- Supports:
- Change notifications
- Selective options invalidation (IOptionsMonitorCache<TOptions>)
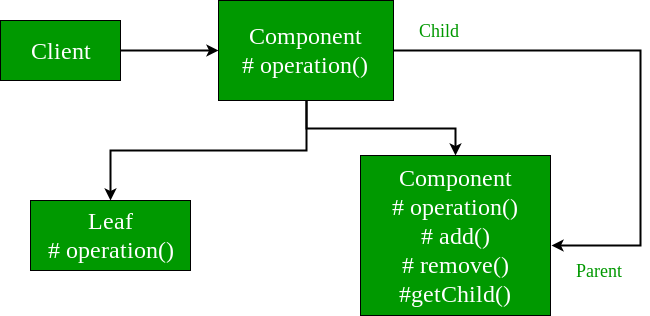
IOptionsFactory<TOptions> is responsible for creating new options instances. It has a single Create method. The default implementation takes all registered IConfigureOptions<TOptions> and IPostConfigureOptions<TOptions> and runs all the configurations first, followed by the post-configuration. It distinguishes between IConfigureNamedOptions<TOptions> and IConfigureOptions<TOptions> and only calls the appropriate interface.
IOptionsMonitorCache<TOptions> is used by IOptionsMonitor<TOptions> to cache TOptions
instances. The IOptionsMonitorCache<TOptions> invalidates options instances in the monitor so that the value is recomputed (TryRemove). Values can be manually introduced with TryAdd. The Clear method is used when all named instances should be recreated on demand.
Options interfaces benefits
Using a generic wrapper type gives you the ability to decouple the lifetime of the option from the DI container. The IOptions<TOptions>.Value interface provides a layer of abstraction, including generic constraints, on your options type. This provides the following benefits:
- The evaluation of the
T
configuration instance is deferred to the accessing of IOptions<TOptions>.Value, rather than when it is injected. This is important because you can consume theT
option from various places and choose the lifetime semantics without changing anything aboutT
. - When registering options of type
T
, you do not need to explicitly register theT
type. This is a convenience when you're authoring a library with simple defaults, and you don't want to force the caller to register options into the DI container with a specific lifetime. - From the perspective of the API, it allows for constraints on the type
T
(in this case,T
is constrained to a reference type).
Use IOptionsSnapshot to read updated data
When you use IOptionsSnapshot<TOptions>, options are computed once per request when accessed and are cached for the lifetime of the request. Changes to the configuration are read after the app starts when using configuration providers that support reading updated configuration values.
The difference between IOptionsMonitor
and IOptionsSnapshot
is that:
IOptionsMonitor
is a singleton service that retrieves current option values at any time, which is especially useful in singleton dependencies.IOptionsSnapshot
is a scoped service and provides a snapshot of the options at the time theIOptionsSnapshot<T>
object is constructed. Options snapshots are designed for use with transient and scoped dependencies.
The following code uses IOptionsSnapshot<TOptions>.
The following code registers a configuration instance which TransientFaultHandlingOptions
binds against:
Can I Wear Pattern On Pattern
In the preceding code, changes to the JSON configuration file after the app has started are read.
IOptionsMonitor
The following code registers a configuration instance which TransientFaultHandlingOptions
binds against.
The following example uses IOptionsMonitor<TOptions>:
In the preceding code, changes to the JSON configuration file after the app has started are read.
Tip
How To Make A Pattern In Art
Some file systems, such as Docker containers and network shares, may not reliably send change notifications. When using the IOptionsMonitor<TOptions> interface in these environments, set the DOTNET_USE_POLLING_FILE_WATCHER
environment variable to 1
or true
to poll the file system for changes. The interval at which changes are polled is every four seconds and is not configurable.
For more information on Docker containers, see Containerize a .NET app.
Named options support using IConfigureNamedOptions
Named options:
- Are useful when multiple configuration sections bind to the same properties.
- Are case-sensitive.
Consider the following appsettings.json file:
Rather than creating two classes to bind Features:Personalize
and Features:WeatherStation
,the following class is used for each section:
The following code configures the named options:
The following code displays the named options:
All options are named instances. IConfigureOptions<TOptions> instances are treated as targeting the Options.DefaultName
instance, which is string.Empty
. IConfigureNamedOptions<TOptions> also implements IConfigureOptions<TOptions>. The default implementation of the IOptionsFactory<TOptions> has logic to use each appropriately. The null
named option is used to target all of the named instances instead of a specific named instance. ConfigureAll and PostConfigureAll use this convention.
OptionsBuilder API
How To Make Paint Patterns
OptionsBuilder<TOptions> is used to configure TOptions
instances. OptionsBuilder
streamlines creating named options as it's only a single parameter to the initial AddOptions<TOptions>(string optionsName)
call instead of appearing in all of the subsequent calls. Options validation and the ConfigureOptions
overloads that accept service dependencies are only available via OptionsBuilder
.
OptionsBuilder
is used in the Options validation section.
Use DI services to configure options
Services can be accessed from dependency injection while configuring options in two ways:
Pass a configuration delegate to Configure on OptionsBuilder<TOptions>.
OptionsBuilder<TOptions>
provides overloads of Configure that allow use of up to five services to configure options:Create a type that implements IConfigureOptions<TOptions> or IConfigureNamedOptions<TOptions> and register the type as a service.
We recommend passing a configuration delegate to Configure, since creating a service is more complex. Creating a type is equivalent to what the framework does when calling Configure. Calling Configure registers a transient generic IConfigureNamedOptions<TOptions>, which has a constructor that accepts the generic service types specified.
Options validation
Options validation enables option values to be validated.
Consider the following appsettings.json file:
The following class binds to the 'Settings'
configuration section and applies a couple of DataAnnotations
rules:
The following code:
- Calls AddOptions to get an OptionsBuilder<TOptions> that binds to the
SettingsOptions
class. - Calls ValidateDataAnnotations to enable validation using
DataAnnotations
.
The ValidateDataAnnotations
extension method is defined in the Microsoft.Extensions.Options.DataAnnotations NuGet package.
The following code displays the configuration values or the validation errors:
The following code applies a more complex validation rule using a delegate:
IValidateOptions for complex validation
The following class implements IValidateOptions<TOptions>:
IValidateOptions
enables moving the validation code into a class.
Using the preceding code, validation is enabled in ConfigureServices
with the following code:
Options post-configuration
Set post-configuration with IPostConfigureOptions<TOptions>. Post-configuration runs after all IConfigureOptions<TOptions> configuration occurs, and can be useful in scenarios when you need to override configuration:
PostConfigure is available to post-configure named options:
Use PostConfigureAll to post-configure all configuration instances:
